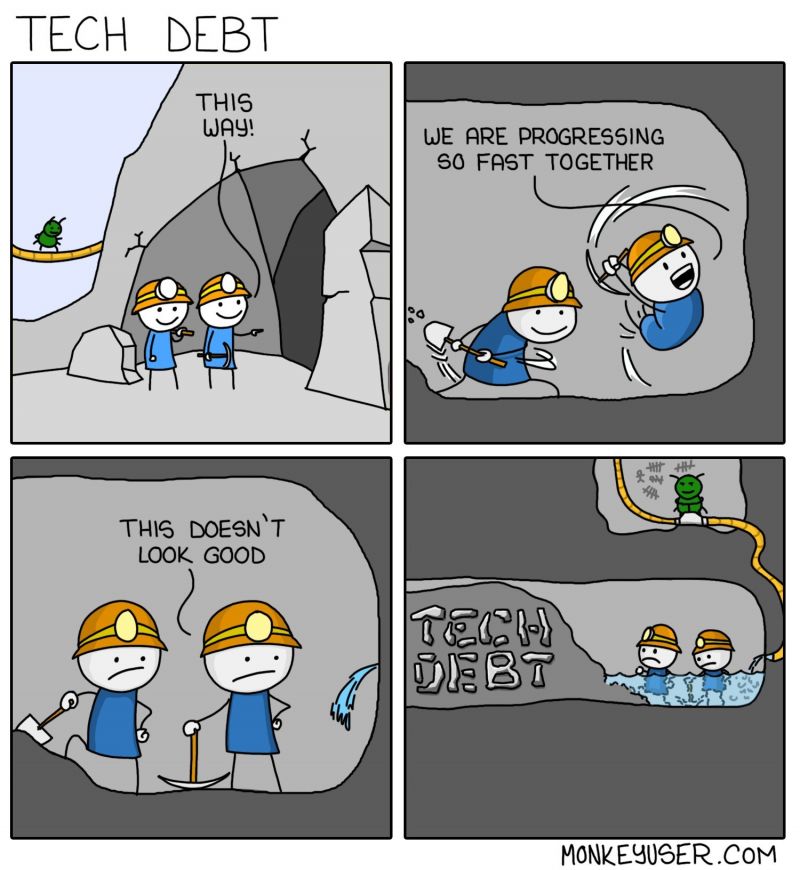
As Java engineers, we live in a world of code. Day after day, we battle deadlines, deal with shifting requirements, and juggle the demands of stakeholders who seem to believe that software development is just a magical process of sprinkling ones and zeros. Somewhere along the way, the beast called technical debt sneaks into our codebase, lurking in dark corners and waiting to grow into a full-blown catastrophe. But what exactly is this debt, and why does it matter so much? Let’s dive into the nitty-gritty with few examples.
What Is Technical Debt?
Technical debt is the programming equivalent of taking out a high-interest loan to buy a sports car because you want it now. Sure, you get to show it around town, but soon enough, the EMI payments start piling up, and you’re left wondering if it was worth the trade-off.
In software terms, technical debt arises when we prioritize speed over quality. It’s that “just get it done” patchwork solution we apply to meet deadlines, knowing full well it’s going to cause headaches later. It’s the hastily written method, the poorly named variable (temp123
, anyone?), or the decision to skip writing tests because “we’ll add them later” (we rarely do).
Imagine you’re building a payment processing system for an e-commerce platform. The deadline is yesterday, so instead of implementing a robust, modular design, you slap together a monolithic block of spaghetti code. It works for now—hooray! But six months later, when a business wants to use cryptocurrency, you’re left crying into your keyboard because the entire payment flow depends on an outdated assumption: “Everything is either a credit card or UPI.”
Why Does Technical Debt Occur?
Technical debt doesn’t just fall from the sky like a Java NullPointerException
in poorly written code. It’s usually the result of human decisions, often influenced by tight schedules, resource constraints, or plain old ignorance.
One major culprit is deadline pressure. You’re working on a Spring Boot project, and your manager walks in with that gleam in their eye that means trouble: “We need this feature live by Friday.” You know it’s going to take a week to build it properly, but against your better judgment, you duct-tape a solution together, promising yourself you’ll clean it up later. Spoiler alert: you won’t.
Another factor is evolving requirements. Let’s say you’ve designed a beautiful REST API for managing user accounts. Then, mid-development, the product team decides users should be able to log in using only via UBS Neo. Your elegant design suddenly feels like trying to fit a square peg into a round hole. Instead of redesigning, you shoehorn the new functionality into the existing system, setting the stage for future disasters.
Sometimes, it’s a matter of skill gaps. Not every Java developer is an expert in clean code principles or design patterns. Maybe a junior developer writes an overly complex algorithm when a simple for
loop would suffice. Or maybe someone new to Hibernate creates a query so inefficient it could crash a supercomputer. These innocent mistakes accumulate over time, creating a mountain of technical debt.
The Factors Driving Technical Debt
Technical debt doesn’t just happen in isolation. It’s a perfect storm of poor practices, bad decisions, and external pressures. Culture plays a massive role. If your organization values speed over quality, you’re more likely to see shortcuts being taken. Lack of proper tooling is another big one. Without the right CI/CD pipelines or code review processes, issues can slip through the cracks.
And then there’s the temptation of legacy systems. Oh, legacy code—the gift that keeps on giving. You know the kind: a monolithic Java application written in 2005 that still uses Java 1.4 syntax because “it works, so why touch it?” Adding anything to such systems is like trying to renovate a crumbling house: every hammer blow reveals another crack.
Avoiding the Trap: How to Prevent Technical Debt
Preventing technical debt is like avoiding bugs in production: it’s not entirely possible, but you can minimize the risk. First, prioritize clean architecture. In Java, this means embracing modularity, adhering to SOLID principles, and using tools like Spring Framework to structure your codebase sensibly. Resist the urge to cut corners, even when deadlines loom. A quick fix today is tomorrow’s nightmare.
For example, imagine building an e-commerce search feature. Instead of embedding search logic directly into a controller, you create a separate service layer and interface. It takes a little longer upfront, but when the marketing team decides they want predictive search with AI, you’ll thank yourself for the clean separation of concerns.
Testing is another lifesaver. As Java engineers, we have robust tools like JUnit and Mockito at our disposal. Use them religiously. Writing tests might feel like a chore, but it’s far less painful than debugging a production failure at 2 a.m. And don’t just stop at unit tests—add integration tests, performance tests, and, if you’re working with APIs, contract tests.
Finally, automate as much as possible. Tools like Jenkins, GitLab CI, and SonarQube can help enforce code quality and catch issues before they make it into production. Think of them as your vigilant sidekick, always on the lookout for trouble.
Cleaning Up the Mess: How to Eliminate Existing Technical Debt
Eliminating technical debt is like decluttering your garage: overwhelming at first but deeply satisfying when done right. Start with a debt inventory. Identify the worst offenders—code that’s buggy, hard to read, or downright unmaintainable. Tools like SonarQube can help by analyzing your Java codebase and pointing out hotspots.
Next, prioritize. Not all debt needs to be paid off immediately. Focus on areas that have the greatest impact on your system’s performance or scalability. For instance, if a poorly written method is slowing down a key API endpoint, fix it before you refactor that obscure helper class no one even uses anymore.
Refactoring is your best friend here. Let’s say you’ve got a 500-line method in a legacy Java project (we’ve all seen them). Break it into smaller, more manageable chunks. Replace hardcoded values with constants or configuration files. Use modern Java features like streams and lambdas to simplify loops and conditionals. It’s like giving your code a much-needed makeover.
Sometimes, the best way to tackle technical debt is to start fresh. This is especially true for systems so riddled with debt that fixing them would take longer than rewriting them. If you’re migrating a monolithic Java application to microservices, take the opportunity to address existing issues rather than blindly replicating them.
The Human Element: Building a Culture That Resists Debt
Addressing technical debt isn’t just a technical challenge—it’s a cultural one. Organizations need to value quality as much as speed. Developers should be encouraged to write clean, maintainable code, even if it means pushing back on unrealistic deadlines.
Knowledge sharing is critical. Senior developers should mentor juniors, teaching them best practices and reviewing their code. For example, a seasoned Java engineer can guide a junior on when to use dependency injection or how to design a robust interface. Pair programming can also help transfer knowledge and catch issues early.
Celebrate good practices. When someone writes a beautifully refactored piece of code or designs a scalable architecture, acknowledge it. Positive reinforcement goes a long way in building a team that cares about quality.
Embracing the Inevitable
At the end of the day, some level of technical debt is unavoidable. The key is managing it responsibly. Think of it like a credit card: a useful tool when used wisely but a potential disaster if you let it spiral out of control.
As Java engineers, we have the skills, tools, and knowledge to tackle technical debt head-on. It’s not always glamorous work, but it’s what separates good software from great software. And when you finally eliminate that last piece of debt-riddled code, you’ll feel like a developer superhero.