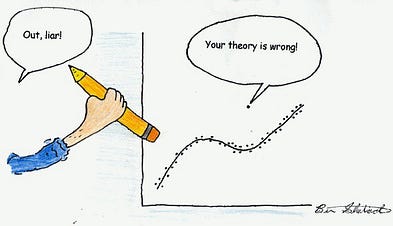
Imagine your house has a really nosy neighbor. This neighbor doesn’t just watch for odd activity; they know everything about your day-to-day. Every morning, they see you sip your coffee at 7:30 a.m., wave as you leave for work at 8:00, and they know that when you start the car, it makes that slightly embarrassing squeak. Now, if one day you were to leave at 2 a.m. wearing a dinosaur costume, they’d probably take notice (and maybe some photos for good measure). This is the essence of anomaly detection: spotting the dinosaur-costume-level weirdness in a sea of normal, squeaky-car routines.
Anomaly detection is like that sharp-eyed neighbor but with data — it’s constantly on the lookout for anything that’s out of the ordinary. It’s the AI-driven method of sniffing out those data points that just don’t belong. In the world of data, anomalies could mean anything from a credit card transaction that seems fishy to a blip in a manufacturing sensor that might signal an imminent machine breakdown. This powerful technique has saved countless organizations from fraud, kept our machines from going kaput, and has even helped catch sneaky hackers.
To make it all work, AI brings its own magic — or should I say, mathemagic. AI has some brilliant algorithms up its sleeve, like clustering, which groups data into clumps of similarity. If you’re anything like most people, imagine clustering as your method for putting all your favorite snacks in one cabinet and hiding them from your roommate (who is the anomaly in this case if they raid it). And when something strange shows up — like, say, a broccoli in your snack cabinet — the system flags it as weird. Another AI friend is the Isolation Forest algorithm. Picture yourself lost in a forest full of squirrels (the normal data), but you’re wearing a shiny suit of armor (the anomaly). Isolation Forest would spot you in no time, because the squirrels are blending in, and you’re not.
And AI isn’t just finding weird broccoli and armored humans — it’s getting good at making sense of these oddballs, too. Deep learning algorithms, especially those mysterious-sounding autoencoders, are like the Sherlock Holmes of anomalies. They can crunch thousands of data points, learning the typical behavior of the data with such finesse that anything that doesn’t fit will trigger a loud mental “Aha!” This is great for catching not just single oddballs, but groups of them, like when your entire fridge decides it’s going to heat food instead of cool it.
Of course, like that neighbor who sometimes calls the cops just because you’re up late binge-watching, anomaly detection isn’t perfect. It can be over-sensitive, triggering false positives and alarming you over what turns out to be nothing more than a stray, harmless squirrel. But like that neighbor, you still want it there because, for every silly false alarm, anomaly detection may just help avert disaster when something genuinely weird goes down.
So the next time you notice your bank account flagging you for buying an out-of-character ₹10,000 worth of instant noodles, or your smartwatch freaking out over an uncharacteristically enthusiastic run to the donut shop, remember: it’s just your AI anomaly detector, keeping a watchful, slightly suspicious eye on things. Whether it’s data patterns or a late-night dinosaur sighting, anomaly detection’s got your back (and probably a few logs to report it).
Long story short, Anomaly detection is a critical task in various fields, including fraud detection, cybersecurity, and predictive maintenance. It involves identifying unusual patterns that deviate from expected behavior in datasets. In machine learning, anomaly detection can be approached through statistical, machine learning, and deep learning methods.
This guide explores the implementation of anomaly detection techniques in Java, using popular libraries like Weka for machine learning algorithms and DeepLearning4J (DL4J) for deep learning-based approaches.
1. Setting Up the Environment
To get started with anomaly detection in Java, we’ll use the following tools and libraries:
- Weka: A collection of machine learning algorithms for data mining tasks.
- DeepLearning4J (DL4J): A deep learning library for Java that supports neural networks and deep learning models.
- Maven: For dependency management.
Dependencies (Maven)
Add the following dependencies to your pom.xml
file:
<dependencies>
<!-- Weka -->
<dependency>
<groupId>nz.ac.waikato.cms.weka</groupId>
<artifactId>weka-stable</artifactId>
<version>3.8.5</version>
</dependency>
<!-- DeepLearning4J -->
<dependency>
<groupId>org.deeplearning4j</groupId>
<artifactId>deeplearning4j-core</artifactId>
<version>1.0.0-M1.1</version>
</dependency>
</dependencies>
2. Understanding Anomaly Detection Techniques
Anomaly detection methods can be categorized as follows:
- Statistical Methods: These involve using statistical properties to define the norm and detect deviations.
- Machine Learning Methods: Algorithms like Isolation Forest, K-means clustering, and One-Class SVM are common.
- Deep Learning Methods: Autoencoders are popular for detecting anomalies, especially in high-dimensional or time-series data.
3. Implementing Anomaly Detection Using Weka
Example 1: Isolation Forest in Weka
Isolation Forest is an unsupervised machine learning algorithm effective in detecting anomalies by isolating observations through random partitioning. Weka’s IsolationForest
class makes it easy to implement.
-
Load Dataset
Download or create an anomaly detection dataset (such as network traffic data or fraud transaction records) and save it in ARFF format. -
Java Code for Isolation Forest
import weka.classifiers.meta.IsolationForest; import weka.core.Instances; import weka.core.converters.ConverterUtils.DataSource; public class IsolationForestExample { public static void main(String[] args) throws Exception { // Load dataset Instances data = DataSource.read("path/to/dataset.arff"); data.setClassIndex(-1); // Set class index to -1 for unsupervised learning // Initialize Isolation Forest IsolationForest isolationForest = new IsolationForest(); isolationForest.setNumTrees(100); // Set the number of trees isolationForest.buildClassifier(data); // Detect anomalies for (int i = 0; i < data.numInstances(); i++) { double result = isolationForest.classifyInstance(data.instance(i)); System.out.println("Instance " + i + " is " + (result == 1 ? "Normal" : "Anomalous")); } } }
In this code:
- We load the dataset using Weka’s
DataSource
class. IsolationForest
is configured with a specified number of trees.- The
classifyInstance
method outputs the anomaly classification for each instance.
- We load the dataset using Weka’s
4. Advanced Anomaly Detection with Deep Learning Using DL4J
Autoencoders are a powerful approach for anomaly detection, particularly with complex datasets. Here, we use DL4J to build an autoencoder that learns normal data patterns and identifies outliers as anomalies.
-
Prepare the Dataset Convert the data into a format suitable for DL4J, such as CSV, and normalize it for improved model performance.
-
Build an Autoencoder with DL4J
import org.deeplearning4j.nn.conf.NeuralNetConfiguration; import org.deeplearning4j.nn.conf.layers.DenseLayer; import org.deeplearning4j.nn.conf.layers.OutputLayer; import org.deeplearning4j.nn.multilayer.MultiLayerNetwork; import org.nd4j.linalg.api.ndarray.INDArray; import org.nd4j.linalg.dataset.DataSet; import org.nd4j.linalg.factory.Nd4j; import org.nd4j.linalg.lossfunctions.LossFunctions; public class AutoencoderAnomalyDetection { public static void main(String[] args) { int inputSize = 10; // Example input size // Configure Autoencoder MultiLayerNetwork model = new MultiLayerNetwork(new NeuralNetConfiguration.Builder() .updater(new Nesterovs(0.01, 0.9)) .list() .layer(0, new DenseLayer.Builder().nIn(inputSize).nOut(64).activation("relu").build()) .layer(1, new DenseLayer.Builder().nIn(64).nOut(32).activation("relu").build()) .layer(2, new DenseLayer.Builder().nIn(32).nOut(64).activation("relu").build()) .layer(3, new OutputLayer.Builder().nIn(64).nOut(inputSize).activation("sigmoid") .lossFunction(LossFunctions.LossFunction.MSE).build()) .build()); model.init(); // Sample Data INDArray input = Nd4j.rand(1, inputSize); // Replace with real data DataSet dataSet = new DataSet(input, input); // Train the model model.fit(dataSet); // Use the autoencoder to detect anomalies INDArray reconstructed = model.output(input); double reconstructionError = input.distance2(reconstructed); if (reconstructionError > 0.1) { // Threshold for anomaly System.out.println("Anomaly detected with error: " + reconstructionError); } else { System.out.println("No anomaly detected"); } } }
In this example:
- The autoencoder network has three hidden layers, with the output layer reconstructing the input.
- The model is trained with sample data.
- Anomalies are detected based on the reconstruction error. Higher errors indicate the data is unusual, and thus likely anomalous.
5. Evaluation Metrics for Anomaly Detection
Evaluating anomaly detection models requires metrics that account for the imbalanced nature of anomalies. Common metrics include:
- Precision: Measures accuracy of anomalies detected out of all detected anomalies.
- Recall: Measures accuracy of anomalies detected out of actual anomalies.
- F1 Score: The harmonic mean of precision and recall.
You can implement these metrics manually or use libraries like Weka or DL4J’s evaluation classes.
6. Real-World Use Case: Anomaly Detection in Network Traffic
A practical use case for anomaly detection is identifying unusual network activity in cybersecurity. Here’s how to structure a solution:
- Data Preprocessing: Convert network traffic data (e.g., packet size, protocol type) into a format suitable for model training.
- Modeling: Use Isolation Forest or an Autoencoder for unsupervised learning.
- Evaluation: Adjust the threshold for anomaly based on false positives/negatives observed during testing.